Introduction
In therealm of web development, ensuring user authentication is a fundamental aspect of building secure applications. ReactJS, with its component-based architecture and efficient state management, offers an ideal platform for implementing robust authentication systems. By leveraging ReactJS, developers can create seamless and secure login experiences, protecting user data and ensuring authorized access to sensitive features. From building login components to integrating with backend APIs and protecting routes, this comprehensive approach will equip developers with the knowledge and tools necessary to establish a secure authentication process in their ReactJS applications.
In this guide, we'll delve into the technical steps involved in managing user
authentication with ReactJS.
Why Should You Manage UserAuthentication With React JS?
Managinguser authentication with ReactJS is essential for securing web applications. ReactJS provides a robust framework for building dynamic user interfaces, making it ideal for implementing authentication features seamlessly. You can join the React JS Full Course to learn managing user authentication with this platform. By managing authentication within ReactJS, developers can ensure a smooth user experience, handling login/logout functionality, access control, and secure communication with backend servers.
Thisapproach enhances security by preventing unauthorized access to sensitive data
or functionalities. Moreover, ReactJS offers state management capabilities, allowing developers to efficiently handle authentication states across components.
Steps To Manage UserAuthentication With React JS
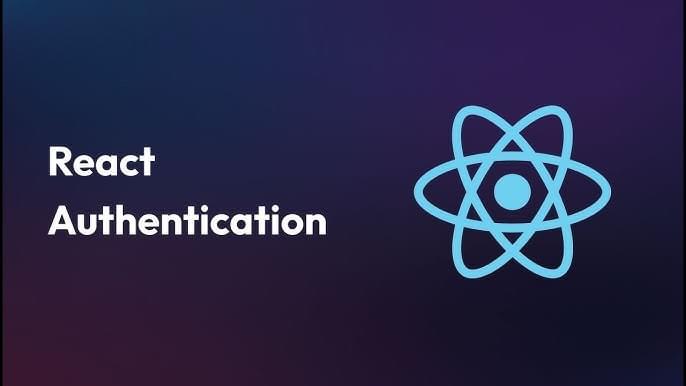
Here's aguide on how to manage user authentication with ReactJS. To begin with, set up a React project with the command “npx create-react-app your-project-name”or “npminstall react-router-dom”. This will help you install the React RouterDom for easy and efficient routing system.
Next, follow the steps below to manage your user authentication with React JS.
- Build Login Component
Start by creating a login component where users can input their credentials. This component should handle form submission and communicate with your authentication logic. Begin by constructing a straightforward login component featuring two input fields: one for the email and another for the password. As users interact with these fields, triggering onChange events, the handleInput function is invoked. Utilizing the name attribute associated with each input, the function manages the state of the respective input field. This method proves efficient for handling input fields within ReactJS components. Upon pressing the button, the parent component listens for an onSubmit event and executes the handleSubmitEvent handler, facilitating the submission of the login credentials. This initial step establishes the foundation for our user authentication process in ReactJS.
Follow thebelow code:
“import {useState } from "react";
const Login= () => {
const [input, setInput] = useState({
username: "",
password: "",
});
const handleSubmitEvent = (e) => {
e.preventDefault();
if (input.username !== ""&& input.password !== "") {
//dispatch action from hooks
}
alert("please provide a validinput");
};
const handleInput = (e) => {
const { name, value } = e.target;
setInput((prev) => ({
...prev,
[name]: value,
}));
};
return (
Email:
type="email"
id="user-email"
name="email"
placeholder="example@yahoo.com"
aria-describedby="user-email"
aria-invalid="false"
onChange={handleInput}
/>
Please enter a valid username. It must contain at least 6 characters.
Password:
type="password"
id="password"
name="password"
aria-describedby="user-password"
aria-invalid="false"
onChange={handleInput}
/>
your password should be more than 6 characters
Submit
);
};
exportdefault Login;”
- Create AuthContext and AuthProvider
The ContextAPI in React is essential for managing state across the application, such as user data or login tokens. In AuthProvider.js, establish an AuthContext to handle user state and an AuthProvider for accessing this context, ensuring seamless integration of user data throughout the application.
Set up an authentication context to manage the authentication state throughout your
application.
“import {useContext, createContext } from "react";
constAuthContext = createContext();
constAuthProvider = ({ children }) => {
return{children};
};
exportdefault AuthProvider;
exportconst useAuth = () => {
return useContext(AuthContext);
};”
The provided code establishes authentication context in React using the Context API. AuthProviderwraps the app, supplying authentication context via AuthContext.Provider. useAuth hook accesses authentication context in components.
Futuresteps involve adding login/logout logic, granting access via AuthProvider, and using them with useAuth function in App.js.
“importAuthProvider from "./hooks/AuthProvider";
functionApp() {
return (
{/* App content*/}
);
}
exportdefault App;”
- Create Authentication Logic
The AuthProvider component is set to receive updates featuring login and logout functionalities. These functions will then be passed down via the AuthContext.Provider, granting global accessibility. Implement the login and logout functions within your authentication logic.
“import {useContext, createContext, useState } from "react";
import {useNavigate } from "react-router-dom";
constAuthContext = createContext();
constAuthProvider = ({ children }) => {
const [user, setUser] = useState(null);
const [token, setToken] =useState(localStorage.getItem("site") || "");
const navigate = useNavigate();
const loginAction = async (data) => {
try {
const response = awaitfetch("your-api-endpoint/auth/login", {
method: "POST",
headers: {
"Content-Type":"application/json"
},
body: JSON.stringify(data),
});
const res = await response.json();
if (res.data) {
setUser(res.data.user);
setToken(res.token);
localStorage.setItem("site",res.token);
navigate("/dashboard");
return;
}
throw new Error(res.message);
} catch (err) {
console.error(err);
}
};
const logOut = () => {
setUser(null);
setToken("");
localStorage.removeItem("site");
navigate("/login");
};
return (
{children}
);
};
exportdefault AuthProvider;
exportconst useAuth = () => {
return useContext(AuthContext);
};”
AuthProvider manages user authentication state, offering login, logout, and token storage via useState hooks. loginAction manages login, sending a POST request, updating user and token state on success, storing token locally. logOut clears user data, removes token from storage. AuthContext.Provider shares authentication state and actions via useAuth hook for component consumption.
- Protect Routes withAuthorization
The nexttask involves implementing a route guard to secure private routes in the application. This requires utilizing the useAuth hook to access context data.Restrict access to certain routes based on authentication status.
Here's thecode to guide you through the process.
“importReact from "react";
import {Navigate, Outlet } from "react-router-dom";
import {useAuth } from "../hooks/AuthProvider";
constPrivateRoute = () => {
const user = useAuth();
if (!user.token) return ;
return ;
};
exportdefault PrivateRoute;”
This code introduces PrivateRoute, leveraging useAuth from AuthProvider to accessuser authentication. If the user lacks a token (not logged in), it redirects to /loginusing . Otherwise, it renders nested child componentsvia , permitting authenticated users access to protected routes,redirecting others to login.
- Add Routing
In theupcoming step, we'll enhance the App.js component by incorporatin grouting into it. Acting as the main component, it envelops the entire application. Within App.js, the routing mechanism is established using the Router component. Set up routing for your application, including public and private routes.
“import {BrowserRouter as Router, Route, Routes } from "react-router-dom";
importLogin from "./components/Login";
importDashboard from "./components/Dashboard";
importAuthProvider from "./hooks/AuthProvider";
importPrivateRoute from "./router/route";
functionApp() {
return (
} />
}>
} />
{/* Other routes */}
);
}
exportdefault App;”
Routes component configures paths: '/login' directs to Login componenton URL match. guards '/dashboard'. Upon '/dashboard' visit, it authenticates via AuthProvider. Authenticated users(with token) access Dashboard; else, redirected to /login, securingdashboard access.
- API Integration
Congratulationson your progress so far! We're close to wrapping up. Now, let's proceed to the next step: initiating the login action. Utilizing the useAuth hook, the handleSubmitEventfunction in the Login Component will trigger the API request. Upon success, tokens and user data will be saved and propagated through the AuthContext. Integrate your authentication logic with backend APIs for user authentication.
Below isthe revised code for the login component.
“import {useState } from "react";
import {useAuth } from "../hooks/AuthProvider";
const Login= () => {
const [input, setInput] = useState({
username: "",
password: "",
});
const auth = useAuth();
const handleSubmitEvent = (e) => {
e.preventDefault();
if (input.username !== ""&& input.password !== "") {
auth.loginAction(input);
return;
}
alert("pleae provide a validinput");
};
return (
{/* Form inputs are provided in the aboveexamples */}
);
};
exportdefault Login;”
- Add Logout Button
Following,we'll integrate a button to dispatch the logOut action, terminating the user session by clearing user state in the context and token from local Storage. Provide users with the ability to log out of their accounts. Additionally, let's create a dashboard component and insert the provided code.
“importReact, { useEffect } from "react";
import {useAuth } from "../hooks/AuthProvider";
constDashboard = () => {
const auth = useAuth();
return (
Welcome!{auth.user?.username}
auth.logOut()} className="btn-submit">
logout
);
};
exportdefault Dashboard;”
TheDashboard component employs the useAuth hook from the AuthProvider to access authentication data. It presents a welcome message featuring the logged-in user's username and a logout button. This button invokes the logOut function to log out the user.
These steps provide a comprehensive guide to managing user authentication within a ReactJS application, covering login, authentication logic, route protection, and more.
Conclusion
Inconclusion, managing user authentication with ReactJS is crucial for developing secure and reliable web applications. By following the outlined steps, developers can create a robust authentication system that ensures user privacy and data integrity. Building a login component, establishing an AuthContext and AuthProvider, implementing authentication logic, protecting routes with authorization, adding routing, integrating with APIs, and including a logout button are fundamental aspects of a comprehensive authentication process within a ReactJS application. The React JS Interview Questions ofteninvolve questions on user authentication management. Therefore, by incorporating these steps, developers can enhance the security, usability, and functionality of their applications, providing users with a seamless and secure experience while interacting with their platform.